Creation and use of Angular 2 services
To avoid writing code twice or more in an Angular-2-Project, there are services. They support the modularity of the Angular-2-project as well. We will show below how to build and include a service with using an Http-service as example. This service should be the interface between data (in .json format) and data processing (the component).
The starting point is a running Angular-2-instance, created either with the Angular 2 Webpack Starter or Angular CLI.
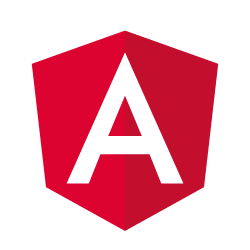
The Service
To begin with, we create a folder named "services" for the HTTPS-service in app/. In this folder you will store a file named "http.service.ts". The following code must initially be inserted into the file:
// http.service.ts
import { Injectable } from '@angular/core';
import { Http } from '@angular/http';
@Injectable()
export class HttpService {
// ...
}
Angular 2 uses Dependency Injection to check if all necessary dependencies are given. So, for the system to check whether the service can be used, we have to install the dependency injection here: This is done by including the injectable module from the Angular core and the @Injectable () command. This command is located directly in the line above the class definition to which it refers.
In addition, we need the Http-module from the Angular Core for an Http-service. This module covers the current Http-methods and provides simplified functions.
In this example, we want to load content from a random JSON-file using the GET-method. In order to do so, the following functions are required in the Http-service:
export class HttpService {
http;
constructor( http: Http ){
this.http = http;
}
getContents(path) {
return this.http.get(path)
.map(response => response.json());
}
}
In the constructor of the service, the Http-module from the Angular Core is available in the service class. With these lines it is possible to access the functions of the module at any time via this.http. This is exactly what is done with the getContents function as well: A path is delivered via the parameter path, which is read using the GET-method of the Http-module. The result is converted into a JSON via response.json. This JSON is now the value of getContents.
Integration of the service into the app
The service currently exists only as a file; it cannot yet be used in other components. To do this, the service must be defined in the app:
// app.module.ts
import { HttpService } from './services/http.service';
In addition, the service must be delivered as a provider of our app. To do so, we add the following entry to @NgModule:
providers: [
HttpService
],
Now we can use the Http-service in the app via dependencies.
It must also be integrated in the component, in which we want to use the Http-service. In this case, our component is called “locations”. In our application the locations.component.ts file is located in app/locations/. It looks like this:
import { Component } from '@angular/core';
import { HttpService } from '../services/http.service';
@Component({
selector: 'locations',
template: `<h1>Locations</h1>`
})
export class Locations {
// Code goes here
}
At first the component-module from the Angular Core is always integrated. The service is then imported with a relative path and without specifying the file type (.ts). Now the methods of the Http-service can be used in the Locations component:
export class Locations {
private locationsList;
constructor( http: HttpService ) {
this.locationsList= http.getContents('/assets/locations.json');
}
}
Here, the/assets/locations.json file is loaded. This is a standard JSON with various entries:
{
"locations": [
{
"id": 1,
"name": "Frankfurt",
"lat": 50.1066202,
"long": 8.6693253
},
{
"id": 2,
"name": "Seville",
"lat": 37.3361456,
"long": -5.9697819
},
{
"id": 3,
"name": "Geneve",
"lat": 46.191196,
"long": 6.1569351
}
]
}
The values can now be displayed and processed further in the component template or in the locations class via locationList.locations.
Conclusion
An Http-service can be very useful for a number of projects, since more and more REST APIs are being used nowadays and the data displayed in the frontend is often delivered as JSON. Within a project an HTTP-request is likely to be executed frequently. Therefore, it is helpful to collect reusable code in one place and create it modularly. With services, this is quite easy in an Angular-2-app. Merely the integration in each component has to be considered.